Laravelで認可を実装してみよう
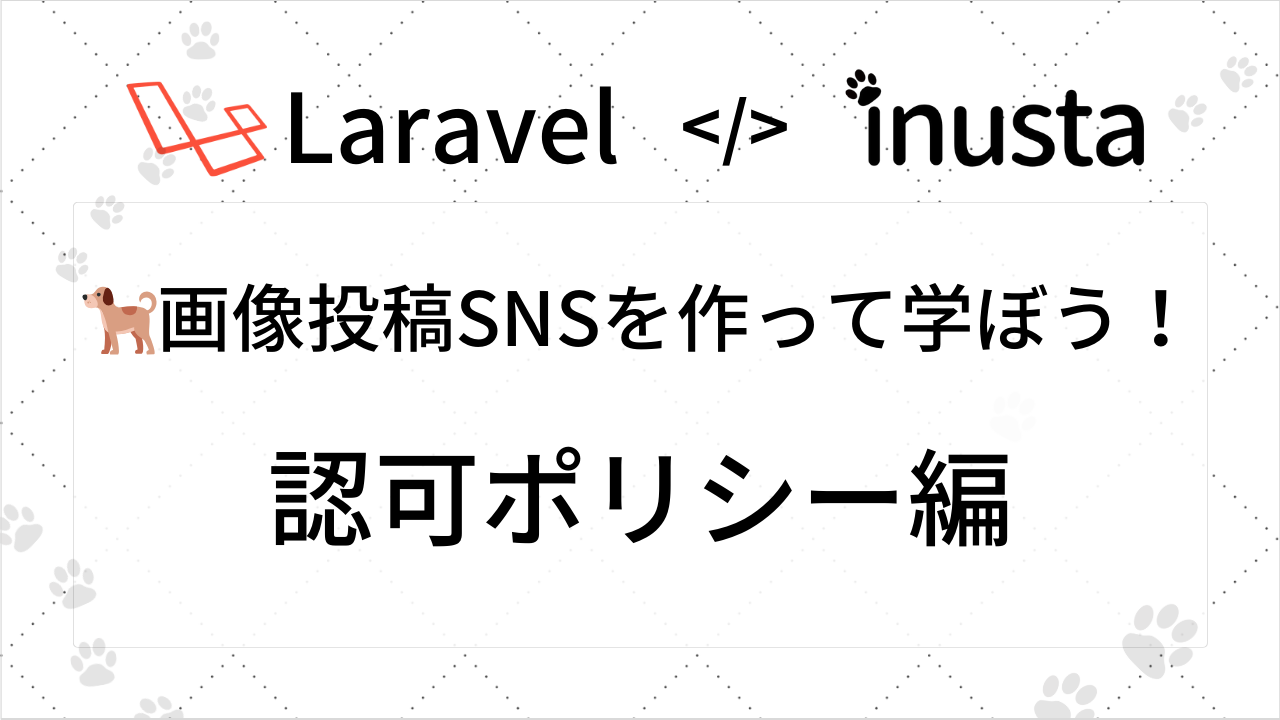
目次
チュートリアルの全体像
本チュートリアルを通じて学べる内容は以下のようになります。
- Laravelの開発環境セットアップ
- Laravel Breezeで認証機能を導入
- Laravelのルーティングを徹底解説
- Laravelのコントーラーを徹底解説
- Laravelのブレイドを使ってみよう
- マイグレーションの仕組みを解説
- シーディングを使ってみよう
- Eloquentの基本と使い方を徹底解説
- 画像アップロードを実装する方法
- バリデーションを実装してみよう
- Laravelで認可処理を実装しよう
動画で学びたい方はこちらから!
解説
認可とは?
認可とは、ある条件を元にリソースに対する特定のアクション権限を許可することです。
ちょっとわかりにくいと思うので、例えば、ブログ投稿システムの例で考えてみましょう。
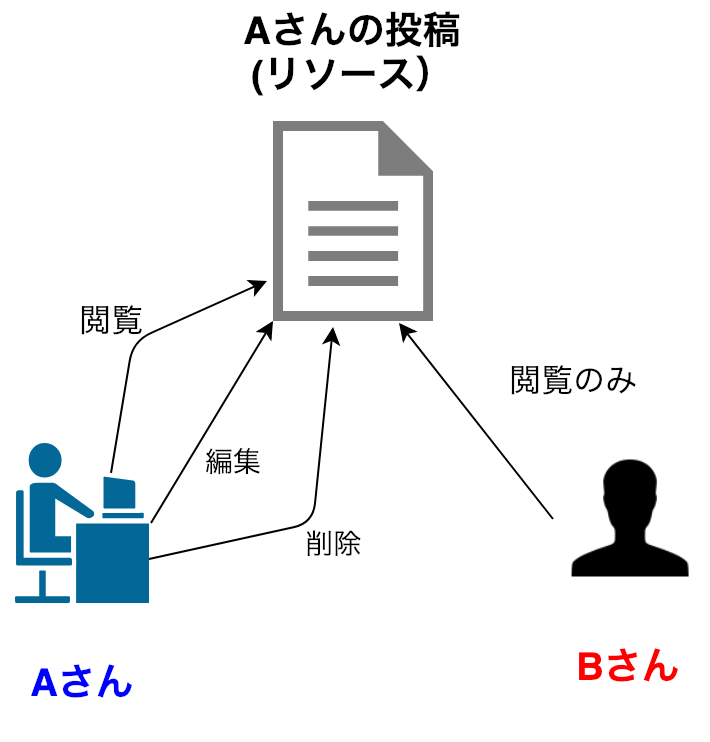
このように、Aさん、Bさん、そしてAさんが投稿した記事(リソース)があります。
もちろん、Aさんの投稿をAさんが編集したり、削除したりするのは自由ですが、Bさんが勝手に編集したり、削除するのはまずいです。
そこで、編集や削除の権限をアプリケーションで制御する必要があるというわけです。さっそく実装していきましょう!
実践
ポリシー作成
php artisan make:policy PostPolicy --model=Post
app/Policies/PostPolicy.php
上記のファイルが作成されます。
ポリシー定義
<?php
namespace App\Policies;
use App\Models\Post;
use App\Models\User;
class PostPolicy
{
/**
* Determine whether the user can view any models.
*/
public function viewAny(User $user): bool
{
return true;
}
/**
* Determine whether the user can view the model.
*/
public function view(User $user, Post $post): bool
{
return true;
}
/**
* Determine whether the user can create models.
*/
public function create(User $user): bool
{
return true;
}
/**
* Determine whether the user can update the model.
*/
public function update(User $user, Post $post): bool
{
return $user->id === $post->user_id;
}
/**
* Determine whether the user can delete the model.
*/
public function delete(User $user, Post $post): bool
{
return $user->id === $post->user_id;
}
/**
* Determine whether the user can restore the model.
*/
public function restore(User $user, Post $post): bool
{
return $user->id === $post->user_id;
}
/**
* Determine whether the user can permanently delete the model.
*/
public function forceDelete(User $user, Post $post): bool
{
return $user->id === $post->user_id;
}
}
フォームリクエスト作成
php artisan make:request DestroyPostRequest
フォームリクエストで認可する
投稿編集
app/Http/Requests/UpdatePostRequest.php
<?php
namespace App\Http\Requests;
use App\Models\Post;
use Illuminate\Foundation\Http\FormRequest;
class UpdatePostRequest extends FormRequest
{
public function authorize(): bool
{
$post = Post::find($this->route('post'))->first();
return $this->user()->can('update', $post);
}
// ...省略...
}
投稿削除
app/Http/Requests/DestroyPostRequest.php
<?php
namespace App\Http\Requests;
use App\Models\Post;
use Illuminate\Foundation\Http\FormRequest;
class DestroyPostRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*/
public function authorize(): bool
{
$post = Post::find($this->route('post'))->first();
return $this->user()->can('delete', $post);
}
// ...省略...
}
フォームリクエストをコントローラに登録
<?php
namespace App\Http\Controllers;
use App\Http\Requests\DestroyPostRequest;
use App\Http\Requests\StorePostRequest;
use App\Http\Requests\UpdatePostRequest;
use App\Models\Post;
use Illuminate\Support\Facades\Storage;
class PostController extends Controller
{
/**
* Store a newly created resource in storage.
*/
public function store(StorePostRequest $request)
{
}
/**
* Update the specified resource in storage.
*/
public function update(UpdatePostRequest $request, Post $post)
{
}
/**
* Remove the specified resource from storage.
*/
public function destroy(DestroyPostRequest $request, Post $post)
{
}
}
この記事へのコメントはありません。